lunes, 11 de enero de 2016
Bluetooth con Arduino y Processing
domingo, 3 de enero de 2016
Arduino ADXL335 - acelerómetro analógico
ADXL335
lunes, 21 de enero de 2013
Prosecessing–Programa para crear aplicaciones para Arduino utilizando el puerto USB.
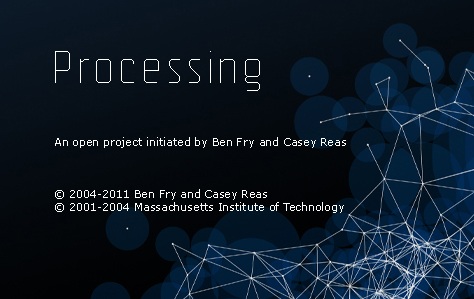
Les presento este programa que para mí es bastante nuevo, pero lo encontré interesante porque se pueden hacer aplicaciones que corran en diferentes sistemas operativos tales como:
Windows
Linux
Mac
Android
Esto lo hace interesante ya que con Visual Basic 2010 solo podrán crear aplicaciones para Windows, aunque las podrían correr en linux utilizando una aplicación que se llama WINE.
Además está acompañado con una lista de ejemplos que podrían utilizar para un rápido aprendizaje, espero les guste ya que la programación es similar a la de los Arduino, de modo que no tendrán que complicarse mucho la vida
.
No sé si ellos tienen una página en español pero no esta demás aprender ingles, les recomiendo que lo intenten los que no dominen el idioma ya que hay muchas herramientas de traducción como, Google Translator, es una de ellas. (http://translate.google.com.pr/?hl=es-419&tab=wT)

La dirección para la página Oficial de Processing (http://processing.org/)
Para bajar el programa (http://processing.org/download/)
Para poder aprender (http://processing.org/learning/)
Espero les guste,
En próximas publicaciones estaremos utilizando este programa comunicándonos con Arduino.
miércoles, 16 de enero de 2013
Fritzing–Disena circuitos para Arduino
Sobre Fritzing
Fritzing es una iniciativa de hardware de código abierto para apoyar a diseñadores, artistas, investigadores y aficionados a trabajar creativamente con la electrónica interactiva. Tutorial Fritzing basico en 12 minutos ESPAÑOL .Descarga y comienza!
http://fritzing.org/home/miércoles, 8 de agosto de 2012
Utilice su PC como osciloscopio gratis.
Un osciloscopio es un instrumento de laboratorio utilizado para mostrar y analizar la forma de onda de las señales electrónicas. Aquí es un procedimiento sencillo, cómo hacer tu propio Osciloscopio para tu PC .
Un osciloscopio real es muy costosa y grande en tamaño, por lo que resulta poco accesible para los aficionados. Podemos utilizar esta alternativa un osciloscopio Virtua para PC .
Encontraras dos modelos que podras utilizar:
Descargar osciloscopio 2,51
Descargar Digital Osciloscopio
Cómo utilizar el osciloscopio
1) Descargue la aplicación y ejecutarlo.
El osciloscopio utiliza la tarjeta de sonido de su computadora (entrada MIC) para detectar señal, así que para proteger su tarjeta de sonido debemos hacer un pequeño circuito de interfaz mediante resistencias. (como se muestra en la figura a continuación)
Utilice cable coaxial para la medición de alta frecuencia, para evitar el ruido
Lista de materiales:-
1) 1 x Cable de Audio de 3.5 a 3.5 macho
2) Resistencias como se muestra en la figura
3) Sondas (puede utilizar sondas de cable multímetro)
4) Coaxial Cable para evitar ruidos durante la medición de señales de alta frecuencia.
Finalmente, tenemos un osciloscopio muy economico para las proyectos.
sábado, 28 de julio de 2012
Arduino LCD indicador de temperatura con LM35
Verdaderamente la plataforma Arduino es muy versátil, y además fácil de comprender. Durante muchos años estuve interesado en los microprocesadores y micro controladores, 8085, Pic, Paralax, Basic Stamp, AVR y otros pero los encontraba muy monótonos, pero cuando me tope con Arduino, me sorprendí, las aplicaciones que se le pueden dar son inmensas y los componentes que se pueden encontrar para esta plataforma, son súper interesantes, los GPS permiten la creación de equipos de vuelo parcialmente autónomos, con tan solo indicar las coordenadas, es un proyecto que más tarde mostrare, con los quadcopter..
También la robótica esta increíble con los Hexápodos, este será otro proyecto que mostrare más adelante, y las aplicaciones en CNC (Fresadoras), en fin son temas que mas adelante tocaremos.
Aqui tienen el sketch para arduino
/*
Temperature Indicator F/C with LM35 sensor.
apcexpert.blogspot.com
apcexpert.wordpress.com
*/
// LCD library code:
#include <LiquidCrystal.h>
// The numbers of the interface pins
LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
// Variables
float TempC;
float TempF;
int TempPin = 1;
void setup(){
// LCD's line position of columns and rows:
lcd.begin(16, 2);
lcd.print(" Temp Indicator");
lcd.setCursor(0, 1);
lcd.print("Temp");
}
void loop(){
TempC = analogRead(TempPin); //read the value from the sensor
TempC = (5.0 * TempC * 100.0)/1024.0; //convertion the analog data to temperature
TempF = ((TempC*9)/5) + 32; //convertion celcius to farenheit
// print result to lcd display
lcd.setCursor(11, 1);
lcd.print(TempC,1);
lcd.print("C");
lcd.setCursor(5, 1);
lcd.print(TempF,1);
lcd.print("F");
delay(1000);
}
Hasta la proxima.
Arduino IR Remote Control - Controlar 4 relays con un control remoto Infrerojo
Hoy les traigo un proyecto de como controlar un panel de 4 relays utilizando in control remoto infrarojo ( IR remote control ). se requiere tener la libreria del sensor infrerojo. Nota importante en esta libreria requiere una pequeña modificacion, solo cuando utilizas las verciones nuevas del programa de Arduino 1.0 y 1.0.1 en las anteriores no tendras problemas.
La modificacion consiste en remplazar #include <WProgram.h>
por #include <Arduino.h>
en el archivo IRRemoteInt.h
.
Si tienen algun problema me abisan, con gusto los ayudare.
Enlace: IR Reomote library
Conecciones electricas:
Pin 13 – canal 1
pin 12 – canal 2
pin 11 – canal 3
pin 10 – canal 4
pin 7 – señal del sensor infrarojo
Estoy utilizando el Control remoto modelo YK-001, lo pueden conseguir en ebay.com, en la imagen podrán ver los códigos de cada tecla, los necesitaran para su proyecto.
Con el siguiente sketch de Arduino podrán localizar los códigos de otros controles, los códigos los podrán ver en el serial monitor, por si desean utilizar otro diferente:
//apcexpert.blogspot.com
// IR Remote Control Code Finder
#include <IRremote.h>
int RECV_PIN = 7; //define input pin on Arduino
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup()
{
Serial.begin(9600);
irrecv.enableIRIn(); // Start the receiver
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value );
irrecv.resume(); // Receive the next value
}
}
Para finalizar, el Sketch de Arduino con el cual podremos controlar los cuatro relay como se ve en la imagen superior;
// apcexpert.blogspot.com
#include <IRremote.h>
int RECV_PIN = 7;
int Relay1_PIN = 13;
int Relay2_PIN = 12;
int Relay3_PIN = 11;
int Relay4_PIN = 10;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup()
{
pinMode(Relay1_PIN, OUTPUT);
pinMode(Relay2_PIN, OUTPUT);
pinMode(Relay3_PIN, OUTPUT);
pinMode(Relay4_PIN, OUTPUT);
pinMode(6, OUTPUT);
irrecv.enableIRIn(); // Start the receiver
}
int on = 1;
void loop() {
if (irrecv.decode(&results)) {
if (results.value == 16724175) { // YK-001 button 1
{
on = !on;
digitalWrite(Relay1_PIN, on ? HIGH : LOW);
}
}
{
if (results.value == 16718055) { // YK-001 button 2
{
on = !on;
digitalWrite(Relay2_PIN, on ? HIGH : LOW);
}
}
{
if (results.value == 16743045) { // YK-001 button 3
{
on = !on;
digitalWrite(Relay3_PIN, on ? HIGH : LOW);
}
}
{
if (results.value == 16716015) { // YK-001 button
{
on = !on;
digitalWrite(Relay4_PIN, on ? HIGH : LOW);
}
}
irrecv.resume(); // Receive the next value
}
}}}}
Espero que no tengan problemas, pero de tenerlos pueden comunicarse y con gusto los ayudare en lo posible. Hasta la próxima.
viernes, 13 de julio de 2012
Arduino–Componentes para control de potencia
Estos son algunos componentes que se podrían utilizar con los proyectos de Arduino, mostrare algunos circuitos básicos para tener ideas de cómo aplicarlos.
1 – Los transistores – la imagen muestra cómo utilizarlos, el transistor debe seleccionarse según el voltaje y la corriente de operación, el especificado en la imagen es el TIP122 este transistor puede ser operado a un máximo de 100voltios y la corriente máxima de operación es de 5 amperios, recuerde que es el máximo, recomiendo utilizarlo al 70% de su capacidad, también aplicar los disipadores de calor.
Especificaciones del Tip122 ( Datasheet ) http://www.fairchildsemi.com/ds/TI/TIP120.pdf
2- Transistores MOSFET- Aunque luce similar al transistor, este no tiene que ver con la corriente que transcurre por su base, es solo el voltaje aplicado le Gate o compuerta de control (similar a la base del transistor), La ventaja con los mosfet es que podemos utilizar motores o cargas con mayor potencia ya que estos pueden manejar corrientes desde 10 hasta 100 amperios, estos son modelos muy comunes también encontraran de mayores capacidades pero son más costosos y no tan accesibles. mostrare algunas imágenes para sus aplicaciones sencillas.
3- Los Solid State Relay( Relevadores en estado sólido) – Esta alternativa lucirá mucho mas profesional si realizan un proyecto comercial o industrial, se utilizan en circuitos de corriente alterna, podrían remplazar los arrancadores magnéticos de motores, sin la necesidad de tener que remplazar contactos como en los controladores magnéticos que se utilizan en las industrias.
Pueden ser controlados con voltajes de 3 hasta 24Voltios DC, son muy versátiles, requieren disipadores de calor y si tienen ventilación será mucho mejor.
Para motores trifasicos
como pueden ver hay diversas formas de contralar cargas desde Arduino. hasta la próxima.
martes, 10 de julio de 2012
Controlar 8 relays con Visual Basic y Arduino
No solo podrán controlar relays, pueden apilarlo en diferentes proyectos como prender motores, lámparas, entre otras cosas. Espero les guste.
Podran utilisar diferentes paneles de relays :
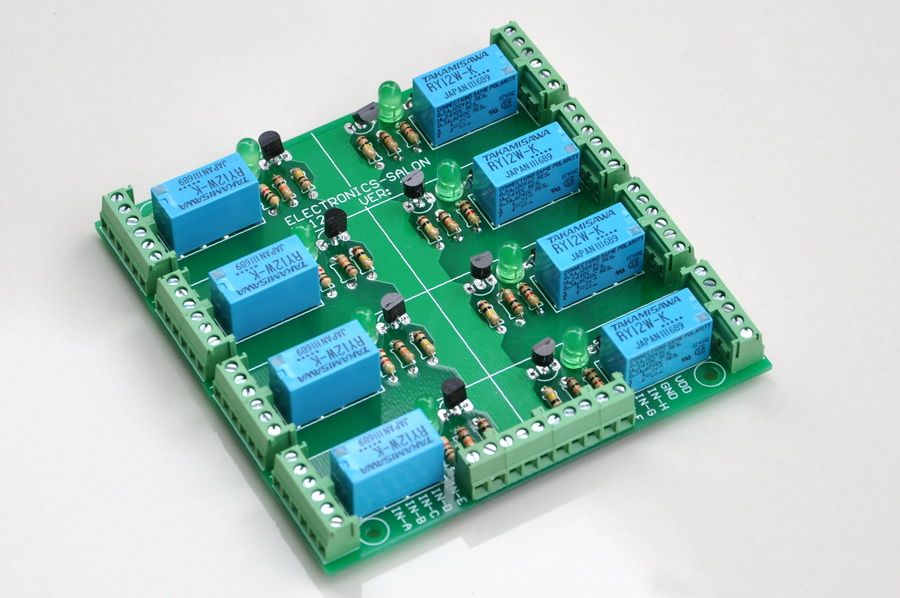
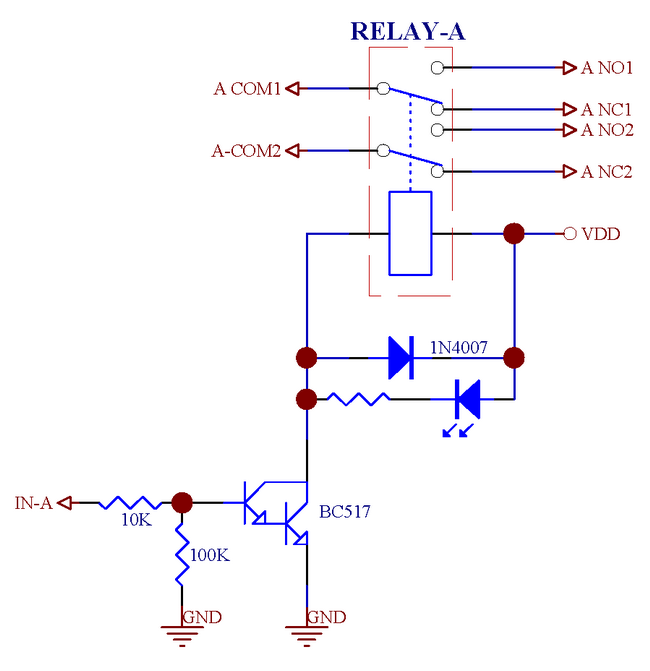
Aquí les dejo el Sketch de Arduino
//apcexpert.blogspot.com
//Con este programa controlaras 8 relays con pulsar un boton para actibar y
//al pursarlo nuevamente se desactiva.
char inData[20]; // Allocate some space for the string
char inChar=-1; // Where to store the character read
byte index = 0; // Index into array; where to store the character
void setup() {
Serial.begin(9600);
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
pinMode(10, OUTPUT);
pinMode(9, OUTPUT);
pinMode(8, OUTPUT);
pinMode(7, OUTPUT);
pinMode(6, OUTPUT);
}
char PinOut(char* This) {
while (Serial.available() > 0) // Don't read unless
// there you know there is data
{
if(index < 19) // One less than the size of the array
{
inChar = Serial.read(); // Read a character
inData[index] = inChar; // Store it
index++; // Increment where to write next
inData[index] = '\0'; // Null terminate the string
}
}
if (strcmp(inData,This) == 0) {
for (int i=0;i<19;i++) {
inData[i]=0;
}
index=0;
return(0);
}
else {
return(1);
}
}
void loop()
{
if (PinOut("13 on")==0) { digitalWrite(13, HIGH);}
if (PinOut("13 off")==0) {digitalWrite(13, LOW);}
if (PinOut("12 on")==0) { digitalWrite(12, HIGH);}
if (PinOut("12 off")==0) {digitalWrite(12, LOW);}
if (PinOut("11 on")==0) { digitalWrite(11, HIGH);}
if (PinOut("11 off")==0) {digitalWrite(11, LOW);}
if (PinOut("10 on")==0) { digitalWrite(10, HIGH);}
if (PinOut("10 off")==0) {digitalWrite(10, LOW);}
if (PinOut("9 on")==0) { digitalWrite(9, HIGH);}
if (PinOut("9 off")==0) {digitalWrite(9, LOW);}
if (PinOut("8 on")==0) { digitalWrite(8, HIGH);}
if (PinOut("8 off")==0) {digitalWrite(8, LOW);}
if (PinOut("7 on")==0) { digitalWrite(7, HIGH);}
if (PinOut("7 off")==0) {digitalWrite(7, LOW);}
if (PinOut("6 on")==0) { digitalWrite(6, HIGH);}
if (PinOut("6 off")==0) {digitalWrite(6, LOW);}
}
Visual Basic 2010
Imports System.IO
Imports System.IO.Ports
Imports System.Threading
Public Class Form1
' Shared _continue As Boolean
' Shared _serialPort As SerialPort
Dim pinout13 As Boolean = True
Dim pinout12 As Boolean = True
Dim pin11 As Boolean = True
Dim pinout10 As Boolean = True
Dim pinout9 As Boolean = True
Dim pinout8 As Boolean = True
Dim pinout7 As Boolean = True
Dim pinout6 As Boolean = True
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
SerialPort1.Close()
SerialPort1.PortName = "com4" 'Cambiar el numero de Puerto "COM"
SerialPort1.BaudRate = 9600
SerialPort1.DataBits = 8
SerialPort1.Parity = Parity.None
SerialPort1.StopBits = StopBits.One
SerialPort1.Handshake = Handshake.None
SerialPort1.Encoding = System.Text.Encoding.Default
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
SerialPort1.Open()
If pinout13 = True Then
SerialPort1.Write("13 on")
RectangleShape1.BackColor = Color.Red
Else
SerialPort1.Write("13 off")
RectangleShape1.BackColor = Color.Lime
End If
pinout13 = Not (pinout13)
SerialPort1.Close()
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
SerialPort1.Open()
If pinout12 = True Then
SerialPort1.Write("12 on")
RectangleShape2.BackColor = Color.Red
Else
SerialPort1.Write("12 off")
RectangleShape2.BackColor = Color.Lime
End If
pinout12 = Not (pinout12)
SerialPort1.Close()
End Sub
Private Sub RectangleShape1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape1.Click, RectangleShape8.Click
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
SerialPort1.Open()
If pin11 = True Then
SerialPort1.Write("11 on")
RectangleShape3.BackColor = Color.Red
Else
SerialPort1.Write("11 off")
RectangleShape3.BackColor = Color.Lime
End If
pin11 = Not (pin11)
SerialPort1.Close()
End Sub
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
SerialPort1.Open()
If pinout10 = True Then
SerialPort1.Write("10 on")
RectangleShape4.BackColor = Color.Red
Else
SerialPort1.Write("10 off")
RectangleShape4.BackColor = Color.Lime
End If
pinout10 = Not (pinout10)
SerialPort1.Close()
End Sub
Private Sub RectangleShape3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape3.Click, RectangleShape6.Click
End Sub
Private Sub RectangleShape4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape4.Click, RectangleShape5.Click
End Sub
Private Sub RectangleShape2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape2.Click, RectangleShape7.Click
End Sub
Private Sub Button8_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button8.Click
SerialPort1.Open()
If pinout6 = True Then
SerialPort1.Write("6 on")
RectangleShape8.BackColor = Color.Red
Else
SerialPort1.Write("6 off")
RectangleShape8.BackColor = Color.Lime
End If
pinout6 = Not (pinout6)
SerialPort1.Close()
End Sub
Private Sub Button5_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button5.Click
SerialPort1.Open()
If pinout9 = True Then
SerialPort1.Write("9 on")
RectangleShape5.BackColor = Color.Red
Else
SerialPort1.Write("9 off")
RectangleShape5.BackColor = Color.Lime
End If
pinout9 = Not (pinout9)
SerialPort1.Close()
End Sub
Private Sub Button6_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button6.Click
SerialPort1.Open()
If pinout8 = True Then
SerialPort1.Write("8 on")
RectangleShape6.BackColor = Color.Red
Else
SerialPort1.Write("8 off")
RectangleShape6.BackColor = Color.Lime
End If
pinout8 = Not (pinout8)
SerialPort1.Close()
End Sub
Private Sub Button7_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button7.Click
SerialPort1.Open()
If pinout7 = True Then
SerialPort1.Write("7 on")
RectangleShape7.BackColor = Color.Red
Else
SerialPort1.Write("7 off")
RectangleShape7.BackColor = Color.Lime
End If
pinout7 = Not (pinout7)
SerialPort1.Close()
End Sub
End Class
Hasta la proxima.
lunes, 9 de julio de 2012
Arduino y Visual Basic 2010–Controlar relay con un solo boton
Lo prepare por una petición en los comentarios y aquí esta la respuesta.
Primero el ejemplo será con 4 Relays y en la próxima publicación será con 8 relays para que puedan ver los cambios realizados y sirva de ejemplo para todos. Espero les guste.
El circuito utilizado será el mismo de la publicación anterior:
Conecciones electricas:
Pin 13 – canal 1
pin 12 – canal 2
pin 11 – canal 3
pin 10 – canal 4
A continuación el Sketch de Arduino UNO
//apcexpert.blogspot.com
//Con este programa controlaras 4 relays con pulsar un botón para activar y
//al pulsarlo nuevamente se desactiva.
char inData[20]; // Allocate some space for the string
char inChar=-1; // Where to store the character read
byte index = 0; // Index into array; where to store the character
void setup() {
Serial.begin(9600);
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
pinMode(10, OUTPUT);
}
char PinOut(char* This) {
while (Serial.available() > 0) // Don't read unless
// there you know there is data
{
if(index < 19) // One less than the size of the array
{
inChar = Serial.read(); // Read a character
inData[index] = inChar; // Store it
index++; // Increment where to write next
inData[index] = '\0'; // Null terminate the string
}
}
if (strcmp(inData,This) == 0) {
for (int i=0;i<19;i++) {
inData[i]=0;
}
index=0;
return(0);
}
else {
return(1);
}
}
void loop()
{
if (PinOut("13 on")==0) { digitalWrite(13, HIGH);}
if (PinOut("13 off")==0) {digitalWrite(13, LOW);}
if (PinOut("12 on")==0) { digitalWrite(12, HIGH);}
if (PinOut("12 off")==0) {digitalWrite(12, LOW);}
if (PinOut("11 on")==0) { digitalWrite(11, HIGH);}
if (PinOut("11 off")==0) {digitalWrite(11, LOW);}
if (PinOut("10 on")==0) { digitalWrite(10, HIGH);}
if (PinOut("10 off")==0) {digitalWrite(10, LOW);}
}
Continuamos con el codigo fuente de Visual Basic 2010
Imports System.IO
Imports System.IO.Ports
Imports System.Threading
Public Class Form1
' Shared _continue As Boolean
' Shared _serialPort As SerialPort
Dim pinout13 As Boolean = True
Dim pinout12 As Boolean = True
Dim pin11 As Boolean = True
Dim pinout10 As Boolean = True
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
SerialPort1.Close()
SerialPort1.PortName = "com4" 'Cambiar el numero de Puerto "COM"
SerialPort1.BaudRate = 9600
SerialPort1.DataBits = 8
SerialPort1.Parity = Parity.None
SerialPort1.StopBits = StopBits.One
SerialPort1.Handshake = Handshake.None
SerialPort1.Encoding = System.Text.Encoding.Default
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
SerialPort1.Open()
If pinout13 = True Then
SerialPort1.Write("13 on")
RectangleShape1.BackColor = Color.Red
Else
SerialPort1.Write("13 off")
RectangleShape1.BackColor = Color.Lime
End If
pinout13 = Not (pinout13)
SerialPort1.Close()
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
SerialPort1.Open()
If pinout12 = True Then
SerialPort1.Write("12 on")
RectangleShape2.BackColor = Color.Red
Else
SerialPort1.Write("12 off")
RectangleShape2.BackColor = Color.Lime
End If
pinout12 = Not (pinout12)
SerialPort1.Close()
End Sub
Private Sub RectangleShape1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape1.Click
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
SerialPort1.Open()
If pin11 = True Then
SerialPort1.Write("11 on")
RectangleShape3.BackColor = Color.Red
Else
SerialPort1.Write("11 off")
RectangleShape3.BackColor = Color.Lime
End If
pin11 = Not (pin11)
SerialPort1.Close()
End Sub
Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
SerialPort1.Open()
If pinout10 = True Then
SerialPort1.Write("10 on")
RectangleShape4.BackColor = Color.Red
Else
SerialPort1.Write("10 off")
RectangleShape4.BackColor = Color.Lime
End If
pinout10 = Not (pinout10)
SerialPort1.Close()
End Sub
Private Sub RectangleShape3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape3.Click
End Sub
Private Sub RectangleShape4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape4.Click
End Sub
Private Sub RectangleShape2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RectangleShape2.Click
End Sub
End Class
Suerte con el proyecto. Gracias
domingo, 8 de julio de 2012
Visual Basic 2010 y Arduino – Servo control con LCD display.
En el post anterior presente como controlar un servo utilizando un Slider en Visual BASIC 2010, ahora les traigo una actualización para mejorar su apariencia y añadir nuevas funciones. Espero les guste, para que les funcione idéntico tienen que instalar un tipo de letra digital si no la tienen los dígitos no lucirán iguales, lo encontraran en el mismo sitio que este archivo. ( 11569_DIGITAL.ttf )
Enlace a la publicación anterior:
Arduino – Visual Basic 2010 Servo control con LCD display
Arduino Sketch
// servo control con LCD display position
#include <LiquidCrystal.h>
lcd.begin(16, 2);
lcd.print("Servo Position:");
myservo.attach(13);
Serial.begin(9600);
}
lcd.setCursor(0, 1);
case '0'...'9':
val = val * 10 + ch - '0';
break;
case 's':
myservo.write(val);
lcd.print((float)val);
val = 0;
break;
}
}
Public Class ServoController
Private serialPort As New IO.Ports.SerialPort
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Try
With serialPort
.PortName = “COM3”
.BaudRate = 9600
.Parity = IO.Ports.Parity.None
.DataBits = 8
.StopBits = IO.Ports.StopBits.One
End With
serialPort.Open()
serialPort.Write(“0s”)
Catch ex As Exception
MsgBox(ex.ToString)
End Try
End Sub
Private Sub TrackBar1_Scroll(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles TrackBar1.Scroll
serialPort.Write(TrackBar1.Value & “0s“)
ProgressBar1.Value = (TrackBar1.Value * 10)
TextBox1.Text = TrackBar1.Value * 10 & (” “)
End Sub
Private Sub Label2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Label2.Click
End Sub
Private Sub TextBox1_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles TextBox1.TextChanged
End Sub
Private Sub ProgressBar1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ProgressBar1.Click
End Sub
End Class
(ServoController2)